17. Letter Combinations of a Phone Number
Difficulty: Medium
Given a string containing digits from 2-9
inclusive, return all possible letter combinations that the number could represent. Return the answer in any order.
A mapping of digits to letters (just like on the telephone buttons) is given below. Note that 1 does not map to any letters.
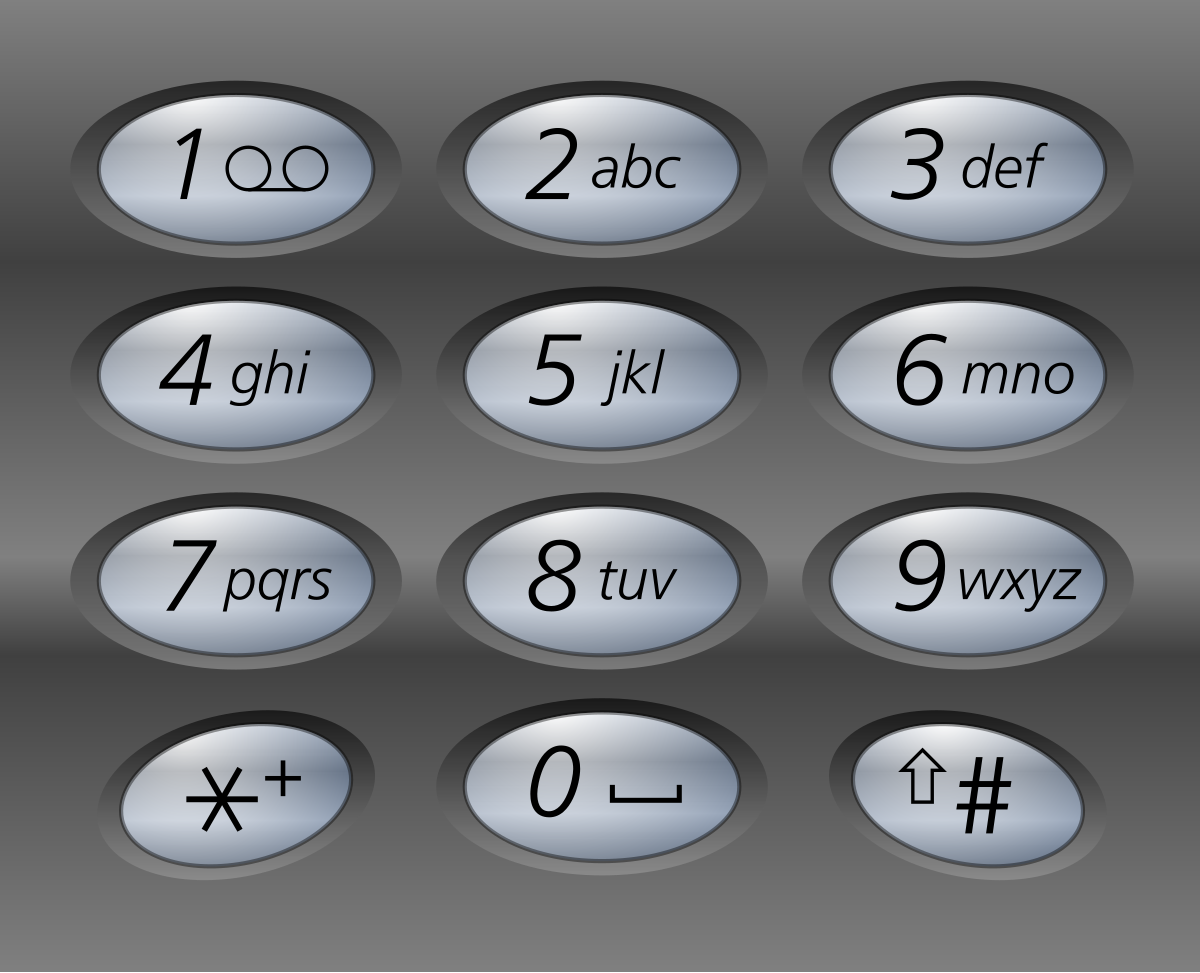
Example 1:
Input: digits = "23" Output: ["ad","ae","af","bd","be","bf","cd","ce","cf"]
Example 2:
Input: digits = "" Output: []
Example 3:
Input: digits = "2" Output: ["a","b","c"]
Python Solution:
class Solution:
def letterCombinations(self, digits: str) -> List[str]:
d={2:'abc',3:'def',4:'ghi',5:'jkl',6:'mno',7:'pqrs',8:'tuv',9:'wxyz'}
i=0
l=[]
try:
for i in d[int(digits[0])]:
try:
for j in d[int(digits[1])]:
try:
for k in d[int(digits[2])]:
try:
for m in d[int(digits[3])]:
l.append(i+j+k+m)
except:
l.append(i+j+k)
except:
l.append(i+j)
except:
l.append(i)
return l
except:
return l
If you have any doubt in code, just ask me in gmail, I'll clear your doubt: varunkapoor.29085@gmail.com
Comments
Post a Comment